As you may aware that all .Net applications are compiled to Microsoft Intermediate Language(MSIL). MSIL maintain a high level information about .net assemblies (exe or dll) such as the code structure that comprises the assembly types and members such as classes, interfaces, properties, methods, fields, etc.
Any hacker or even average person can use a free decompiler such redgate's reflector and ILSpy tools to reverse engineer the process and gain access to your code. Imagine how bad is it if your company invest a lot of money until they ship their grand-award winning product then a competitor just within a couple of hours gain access to your full featured product code. that's sucks right?
Dotfuscator is one of most well-known and reliable obfuscation tool you can either download the free community edition with limited feature or purchase the full professional edition. it makes it difficult against reverse engineering tools to gain access to your code to bypass a license or tampering the product and resell it. as per Preemtive Solutions the creator of Dotfuscator
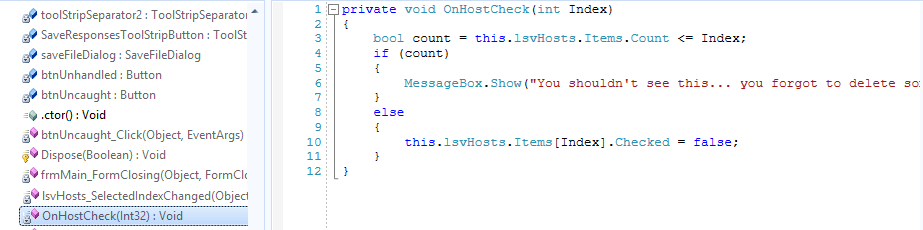
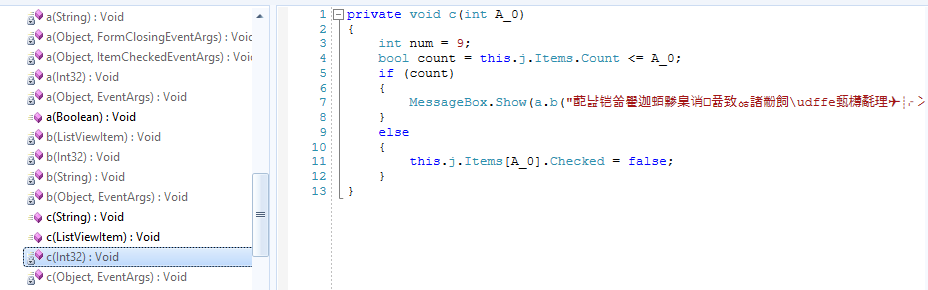
Any hacker or even average person can use a free decompiler such redgate's reflector and ILSpy tools to reverse engineer the process and gain access to your code. Imagine how bad is it if your company invest a lot of money until they ship their grand-award winning product then a competitor just within a couple of hours gain access to your full featured product code. that's sucks right?
Dotfuscator is one of most well-known and reliable obfuscation tool you can either download the free community edition with limited feature or purchase the full professional edition. it makes it difficult against reverse engineering tools to gain access to your code to bypass a license or tampering the product and resell it. as per Preemtive Solutions the creator of Dotfuscator
How does Obfuscation and Code Protection Work?
Various different techniques that complement each other are used to create a layered defense to make reverse engineering, tampering, debugging much more difficult. Some examples include:
- Rename Obfuscation
- String Encryption
- Control Flow Obfuscation
- Unused Code and Metadata Removal
- Binary Linking/Merging
- Dummy Code Insertion
- Instruction Pattern Transformation
- Opaque Predicate Insertion
- Anti-Tamper Wrapping
- Anti-Debug Wrapping
- Root Check for Xamarin.Android
- Watermarking
- Security Alerts
- Custom Response to Tamper or Debugging
Code Before and After Rename Obfuscation and String Encryption Only
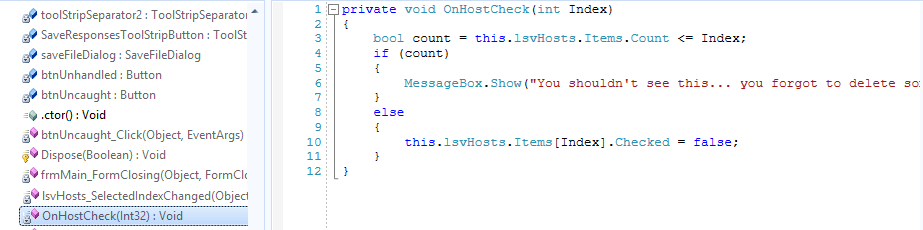
.NET Code Before Obfuscation
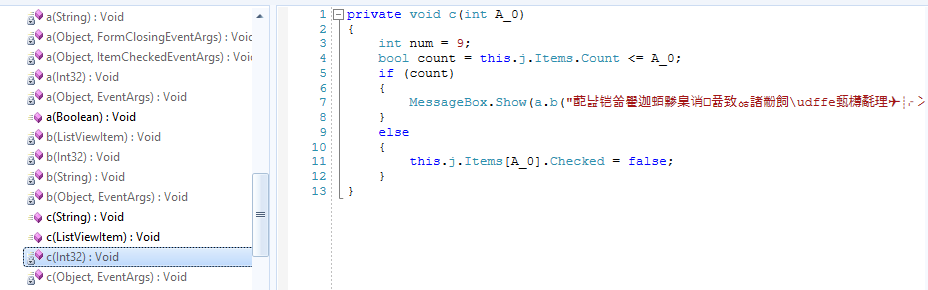
.NET Code After Baseline Obfuscation
Here are the documentation on how to start use the protection tools using GUI and the command line interface.